If you are reading user input using Java’s Scanner class, you might find that the next(), nextInt(), nextDouble(), etc., is followed by the subsequent nextLine() seemingly getting skipped. It is a common pitfall for Java newcomers and usually results in truncated or incorrect input processing.
So what is the cause of the problem? Why does nextLine() act differently after calling other Scanner methods? And more significantly, how do you rectify it? In this article we’re going to discuss:
- The internal workings of Scanner input methods
- Why nextLine() is avoided after next() or nextFoo()
- Step-by-step solutions and code samples on how to manage it correctly
Table of Contents:
What is Scanner in Java?
The Java Scanner class is a built-in facility for reading input from sources such as the standard input of a console, a file, or a stream. It is a part of the java.util package and was introduced with Java 5 (Java SE 1.5). It makes the parsing of primitive types and strings with the help of regular expressions a straightforward process.
Using Scanner, Java programmers can read a variety of inputs using methods such as:
- next() – reads the next token (word) as a string
- nextInt() reads the next token as an int
- nextDouble() – reads the next token as a double.
- nextLine() – reads a whole line of text
Understanding the Scanner Input Problem in Java
It is common for the Scanner class next() and nextFoo() methods, where Foo is any primitive data type like Int, Double, etc., to skip a newline character in the input because these methods are made to read only one token in the input. A token is defined as a sequence of characters that does not include any white space characters.
When the Scanner reads a token using one of the next() or nextFoo() methods, it reads all the characters in the token, including the white space characters that come after the token, but not including any of the white space characters that come before the token.
If you have already used a next() or nextFoo() method to read a token from the input and want to read the rest of the line, you can call the nextLine() method immediately after the next() or the nextFoo() method.
What’s the difference between next() and nextLine()?
The next() Method: The next() method in Java is found in the Scanner class. It is used to get input from the user. To use this method, a Scanner object has to be created. This method reads the input until a space(” “) is found.
The nextLine() Method: It is used to get input from the user. This method reads the input until the end of line, i.e., it takes input until the line changes or a new line comes and ends the input when it gets the ‘n’ or the user presses enter.
In Java, next() and nextLine() have different methods for reading the input.
Example:
Input:
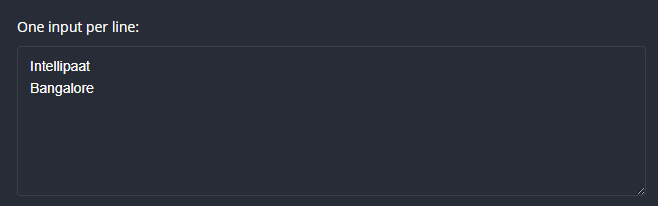
Output:

Explanation: In the above code, when the next() method is called before the nextLine() method, the newline character left in the buffer, which causes the nextLine() to be skipped. To solve this, an extra scanner.nextLine(); should be used after the next() to read the leftover newline in the buffer which will ensure that the nextLine() will work correctly for multi-word input.
Master Java Today – Accelerate Your Future
Enroll Now and Transform Your Future
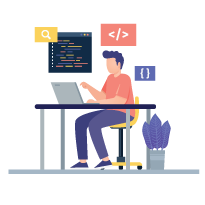
Key Differences between the next() and nextLine() method in Java:
Feature | next() | nextLine() |
---|---|---|
Reads | A single word (token) | The entire line, including spaces |
Stops at | Space, tab, or newline | Newline (n) |
Best for | Single-word input (e.g., first name) | Multi-word input (e.g., full address) |
Example Input | “John Doe” → Reads only “John” | “John Doe” → Reads “John Doe” |
Why can’t You Use nextLine() or next() after nextInt() in Java?
The nextLine() method in Java’s Scanner class is not understood by the user; the way it treats the input is different from the other Scanner methods like nextInt(), next(), and nextDouble().
The problem comes when it is used after the other Scanner methods like next(), nextInt(), nextDouble(), etc.
Understanding the Issue
- The nextLine() method reads all characters on the current line, including the newline character (n).
- Other Scanner methods like nextInt() and next() do not read the newline character left in the buffer.
- As a result, nextLine() may be seen to be “skipped” by the user input.
Example:
Input:

Output:

Explanation of the Fixed Code: Now, the above Java program correctly handles the input problem as it is consuming the leftover newline character (n) after calling scanner.nextInt(). This makes sure that the scanner.nextLine() waits for the user’s input.
1. User Input
Enter an integer: 5Enter your name: John Doe
2. Flow of the program
- When scanner.nextInt() is called, it reads the integer, so nextInt() reads only 5 but leaves the newline (n) in the buffer.
- When scanner.nextLine() is called, it consumes the leftover newline. This removes the newline (n) left after nextInt().
- When scanner.nextLine() is called, it correctly reads the full name. Now, nextLine() waits for the user input instead of reading the leftover newline.
Solutions to Prevent Scanner from Skipping nextLine()
1. Consume the Leftover Newline
After using the nextInt(), nextDouble(), or next() method, explicitly read the newline character by using an extra nextLine() method.
Example:
Input:
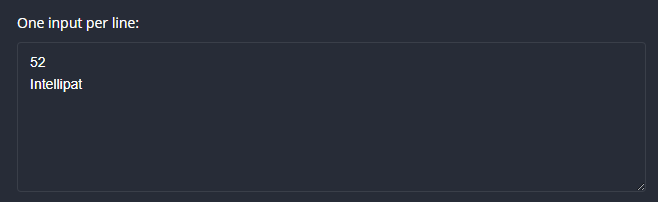
Output:

Explanation: The above Java program reads a number and a name from the user. Since nextInt() doesn’t consume the newline character, nextLine() is used afterwards to clear it which prevents the input from skipping and ensures that the name is read correctly.
2. Read the Whole Line and Parse the Input
Instead of using nextInt(), read the full line using nextLine() and then convert it to an integer by using the Integer.parseInt() method.
Example:
Input:
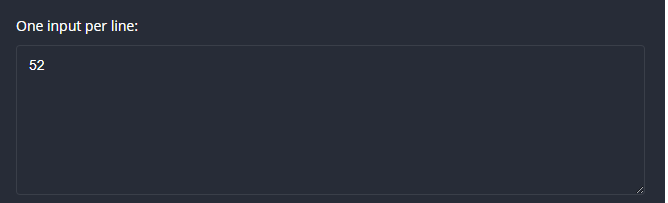
Output:

Explanation: The above Java program is used to read a number from the user using the Scanner class. Instead of using the nextInt() method, it is reading the whole line with the nextLine() method and is converting it to an integer using Integer.parseInt(). This helps prevent nextLine() from getting skipped due to leftover newline characters. Finally, it prints the number and closes the Scanner to prevent resource leaks.
3. Use Only nextLine() for All Inputs
Another approach is to use nextLine() for everything (including numbers) and manually parse them. This completely avoids the issue.
Example:
Input:
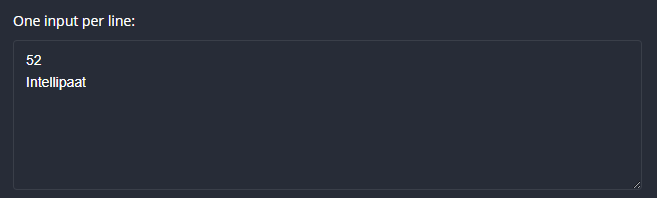
Output:

Explanation: This program ensures smooth input handling by reading a number as a string, converting it to an integer, and accepting the user’s name using nextLine(). By using nextLine() for all inputs, it prevents skipping issues. Finally, it displays the user’s name and number.
Unlock Your Future in Java
Start Your Java Journey for Free Today
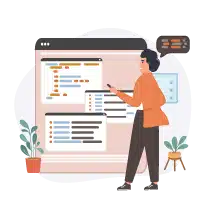
Delimiters and String tokenization
In Java, delimiters and string tokenization play an important role in the way the next() and nextLine() methods take the input.
The next() method uses the whitespaces like spaces, tabs, or newlines as its delimiter. They read the input word by word and stop at the first space, which means that if the user enters “John Doe,” then next() will read “John,” and will leave the “Doe” in the buffer.
Whereas the nextLine() method treats the newline character (n) as its delimiter and reads the entire line as a single string. To use the custom delimiter, the scanner class has the useDelimiter() method, which can be used to define the separators like commas or semicolons.
For example, by using the scanner.useDelimiter(“,”) will allow reading the input and split it by commas instead of the spaces, making it useful for parsing structured data like CSV files.
Example:
Output:

Explanation:In the above code, the delimiter is set to the “,” (comma) using the scanner.useDelimiter(“,”);, method, which allows the input to be split on the basis of commas instead of spaces. The next() method is used to read each token until a comma comes, while (scanner.hasNext()) ensures all tokens are processed. But unlike the nextLine() method, which reads the entire line as a single string. For example, if the input is given as “apple, banana, grape, orange”, the next() takes “apple”, then moves to “banana”, and continues until all tokens are read.
Best Practices for Using a Scanner.nextLine() After Other next() Methods
1. Always Consume the Leftover Newline After next(), nextInt(), etc.
Since methods like nextInt(), nextDouble(), and next() do not read the newline (n), it’s best to use the scanner.nextLine(); right after using them.
2. Use the nextLine() method for Multi-Word Input
If the user needs to enter a full sentence like an address or name with spaces, use the nextLine() instead of next().
3. Use nextLine() for Mixed Data Types
When reading both numbers and strings, it’s safer to use the nextLine() and then parse the numbers.
4. Use Custom Delimiters When Needed
If input is separated by the commas or other characters, set a custom delimiter instead of the delimiter, i.e., spaces.
5. Always Close the Scanner
Closing the Scanner after use frees system resources.
Note: Avoid closing Scanner(System.in) if reading input multiple times, as it will close System.in and prevent further input.
Get 100% Hike!
Master Most in Demand Skills Now!
Conclusion
From the above, we conclude that the Scanner class skips the nextLine() method after the next() or nextFoo() because these methods do not read the newline (n) character, which forces the nextLine() to pick up the remaining newline instead of waiting for input from the user. To solve this, the scanner.nextLine(); method should be added after nextFoo(). Knowing the difference between next() and nextLine(), using custom delimiters, and following best practices can prevent input issues.
If you want to learn more about Java, you can refer to our Java Course.
Why is the Scanner skipping nextLine after using next or nextFoo – FAQs
Q1. What is the difference between the next() and the nextLine() method?
The next() method reads the input until a whitespace is encountered, whereas nextLine() reads input until a newline character is encountered.
Q2. What does the Scanner nextLine() do?
The nextLine() method returns all text up to a line break.
Q3. Does nextLine return a string?
The nextLine() method returns a string containing all of the characters up to the next new line character in the scanner or up to the end of the scanner if there are no more new line characters.
Q4. How do I skip to the next line in Java?
In Java, n is an escape sequence that represents a newline character. It is used to insert a line break or start a new line in strings or output.
Q5. What happens if we use nextLine() after the nextInt()?
The nextInt() method only consumes the integer part and leaves the enter or newline character in the input buffer. When the third scanner. nextLine() is called, it finds the enter or newline character still existing in the input buffer, mistakes it as the input from the user, and returns immediately.
Q6. What is r in Java strings?
r moves the cursor to the beginning of the line.