Do you know how strings work in C++? Think again. What if the true nature of strings is not in the whole, but in parts? The substr() function in C++ doubles as a powerful tool for unlocking hidden patterns, parsing through complex data, and decoding convoluted algorithmic challenges. However, are you using these substrings properly? In this article, you are going to learn the concept of substrings in C++, from its basic syntax to real-world applications.
Table of Contents:
What is a Substring in C++?
In C++, a substring is defined as a subpart of a large string. The substr() function is a member of the std::string class that allows you to extract substrings from a given string, and also specifies the starting position and length of the substring. The substring is part of the std::string class. This member function helps developers with text manipulation and data parsing.
Syntax:
string substr(size_t pos = 0, size_t len = npos) const;
The substr() function returns a portion of the new string from the original string. Pos parameter specifies the starting index of the first character included in the substring. The len parameter specifies the number of characters to be extracted from the original string. Size_t is an unsigned integral type.
Parameters of substr() in C++ String
The substr() function takes two parameters:
- pos: This parameter defines the starting position of the string and must be within the bounds of the original string.
- pos == string.length(), substr() return an empty string.
- pos > string.length, substr() function throws an out-of-range error.
- len: it defines the length of the string; string::npos is the default value of len, i.e., till the end of the string.
Example:
Output:

The above C++ program uses the substr() function for extracting a specified part of a substring. Here, the string “Hello, World!” is the original string, using text.substr(7, 5) to obtain the substring from the starting index at 7, spanning up to 5 characters. This results in getting the substring “World”.
Complexity of Substring in C++
The time complexity of the substring in C++ is O(len), where len refers to the number of characters to be copied.
How does substr() in C++ Work?
We use the substr() function to extract the substring from the original string. When substr(pos, len) is called from the pos index in the original string, it first starts copying characters. The len parameter specifies the number of characters to obtain from a specified string. The substr() function returns the substring that is extracted from the original string by starting at the specified position and continuing for the given number of characters.
Return Value of substr() in C++
In C++, the function substr() returns the new string that is obtained from the extracted substring. The return values are mostly stored in another string or may be used directly in expressions.
Example for substr() in C++
Let’s check some examples that use the substr() function in C++:
1. Substring After a Character
In C++, the “substring after a character” extracts the portion of the string that comes after a specific character. By using the find(), you can easily find the position of the character, and following the substr(), you can get the starting substr just after it. This is essential for tasks like email parsing, URLs, etc.
Example:
Output:

The above C++ program demonstrates the usage of substr with find(). Here, find() is used to locate the ‘@’ in the string, then it uses the substr() function to get everything after it.
2. Substring Before a Character
Here, we will define the string that is before the special character. In C++, you can use the find() function to obtain the position of that character and then apply substr(0, pos) to retrieve everything before it. This is often used to substring the username from an email address.
Example:
Output:

The above C++ program extracts the portion that is before the ‘@’ symbol. It first uses the find() to locate the ‘@’ and uses the substr(0, pos) function to get the actual username. The final result, “user,” is printed on your console.
3. Print All Substrings
It generates and prints all possible substrings of the given string. In C++, this is often accomplished with two nested loops: The first for positioning the beginning and the latter for setting the length. This technique is useful for searching, analyzing, and matching patterns within a string.
Example:
Output:
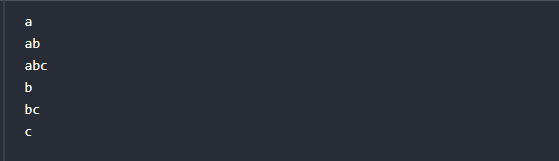
This C++ code will print all the substrings of the string abc. It utilises two nested loops: the outer loop (i), where the starting index of the substring is set, and the inner loop (j), where the length of the substring starting from that index is fixed. We use the substr(i,j) function to create and print every substring. For example, for “abc”, the output would be: “a”, “ab”, “abc”, ”b”, “bc”, “c”.
4. Get Maximum and Minimum Values of All Substrings Representing a Number
This approach refers to extracting all valid substrings from a given string of digits and returning the largest and smallest ones. An approach for this in C++ is to loop through all possible substrings, converting to integers using stoi() and comparing the values to keep track of the minimum and maximum. It comes in handy when dealing with problems involving numeric analysis or pattern identification.
Example:
Output:

This C++ program extracts the maximum and minimum numeric values from all substrings of the string “8347.” It uses nested loops to generate each possible substring, converts it to an integer using stoi(), and then updates the max and min values. As an output, it displays the maximum and minimum formed by the digits of any string.
Detect Overlapping Substrings Using substr() in C++
In a string, overlapping substrings occur more than once in the same string and might share some characters. In contrast to simple repetition, overlapping means that the end of one instance may overlap the beginning of another.
Explanation:
- Accepts a primary string and a target substring.
- Iterates over the primary string with a loop.
- Compare at each index a substring of length equal to the target.
- Advances the index 1 position to enable overlapping.
Example:
Output:

The sample input is a text and a pattern, and we have to find the overlapping substrings in the text; This pattern would solve the problem for us. If so, it increments the counter. In the “ababab” string, “ab” was matched at positions (0, 2, 4), and the return count is 3. The program then prints the total count.
Applications of substr() in C++
- CSV or TSV parsing – A common pattern in most data processing, where strings are split by commas or tabs, and separate fields need to be extracted.
- Log File Analysis – Parse structured log lines to get timestamps, log levels, and messages.
- Often used to generate diagnostics, summaries, or shortened forms of longer strings to display in user interfaces.
- Data Masking – Using splitting and substitution at a certain part of sensitive values, like credit card numbers or phone numbers.
- Longest Palindromic Substring - Determines if a given substring is a palindrome.
- Sliding Window Technique – In a loop, it creates fixed-size substrings useful for problems concerning pattern searches or hashing.
Conclusion
String handling is an important feature in C++, and the substr() function is a great way to create substring subsets of your strings. Use cases like data parsing, text processing, and algorithmic problems. A substr() simplifies and optimises string manipulation, ranging from simple string slicing and trimming to complex tasks, such as log analysis and pattern matching. Mastering substr() is essential for efficient string manipulation in modern C++ applications.
Substring in C++ – FAQs
Q1. What does the substr() function do in C++?
It copies part of a string starting at a given position, with an optional length.
Q2. What happens if the length parameter is not provided in substr()?
The substring will include all the characters from the start index to the end of the string.
Q3. Can substr() throw an exception in C++?
Yes, it can throw std::out_of_range when the start index is greater than the string length.
Q4. Is substr() zero-based in indexing?
Yes, substr() uses zero-based indexing for the start position.
Q5. Can substr() be used with string literals directly?
No, substr() cannot be called directly on a string literal. You must first convert it to an std::string.