HashMap<Key, Value> is a part Java Collection Framework and part of java.util package. It holds the data in the form of Key and Value pairs, where the keys must be distinct. You can sort a Map<Key, Value> according to its values in Java, but there is no direct method to sort it by values.
In this article, we will discuss the methods that can be used to sort a map by its values.
Table of contents:
What is a Map in Java?
In Java, a Map is a collection of values in key-value pairs. However, the entries in a Map are not ordered by default. If you want to sort the map by its values (not by its keys), then you have to do an additional step, as the Map itself does not contain a sort by values.
To sort the values of a map in Java, you can transform the map into a list, sort the list by values, and then store the sorted entries into a map. This is one of the easiest methods, further, there are many other ways you can do it.
Now, before we explore how to sort a Map by its values, let us discuss some of the methods of the Map in Java.
HashMap | Time Complexity |
put | O(1) |
remove | O(1) |
get | O(1) |
containsKey | O(1) |
The space complexity of HashMap is O(n), where n is the number of entries in the map.
Java Certification Training Course
This is comprehensive training course in Java programming language that will make you grow in your software coding career.
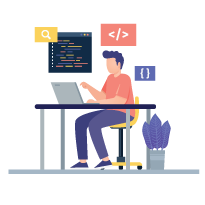
Different Methods to Sort a HashMap by Values in Java
There are several ways to sort a Map<Key, Value> by its values in Java. Some of them are as follows:
1. Using List<Map.Entry<K, V>> and Comparator in Java
This is the most common and simplest method to sort a map by its values. It requires converting the Map values into a list, sorting the list, and then putting it back into a map.
Steps to sort values using a list:
- Convert the map values to a list.
- Sort the list using a custom comparator that compares the values.
Example:
Output:

Explanation: In the above Java code, the values of the Map are converted into a List. Then the List is sorted by the values using a custom comparator. After that, the sorted entries are added into the LinkedHashMap. And finally, it is printed.
2. Using Stream API in Java
The Stream API was introduced in Java 8, and it processes the data in a functional style. It has operations like filtering, mapping, and sorting that can be used with the Collections. When working with a Map, we convert the collections into a stream of entries first, so that we can apply stream operations like sorting on it.
Steps to Sort the Values using a Stream API:
- You can use the entrySet() method to convert the map into a stream of key-value pairs.
- Now, sort the values using the sorted() method, and then we pass it in a comparator that compares entries by their values.
- After that, use the collect() method to collect the sorted entries into a LinkedHashMap to maintain the order of the values.
Example:
Output:

Explanation: In the above Java code, the Stream API is used to convert the map’s entries into a stream, then sort them by values. After it collects the sorted entries into a LinkedHashMap to maintain the order. The result is a sorted LinkedHashMap with entries by their values.
3. Using Lambda Expressions in Java
A lambda expression is a short way to write a function in Java. They allow you to create anonymous functions, i.e., short pieces of code that can be used.
Steps to sort values based on a lambda expression:
- First, convert the Map entries into a stream using the entrySet() method, which will return a set of key-value pairs.
- Now, sort the stream generated in the above step using a lambda expression. We will use the sorted() method and a lambda expression that will compare the map entries by their values.
- Finally, we can collect the sorted entries into a LinkedHashMap.
Example:
Output:

Explanation: In the above Java code, the lambda expression is used to sort a Map by its values. First, it compares the map values using the lambda expression. Then these entries are collected into a LinkedHashMap so that the order is maintained.
4. Using sort() Method in Java
This method uses the sort() method to sort the Map entries by values. As the Map itself does not maintain an order. The sort() method can be used to sort the Map values in Java.
Steps to sort the values using a sort() method:
- Convert the Map entries into a List: Since a Map does not support sorting directly, we will first convert the Map entries into a list of Map.Entry<K, V> objects.
- Use the sort() method: Once we have the Map values in a list, we can use the sort() method of the List interface or Collections.sort() method to sort the values.
Example:
Output:
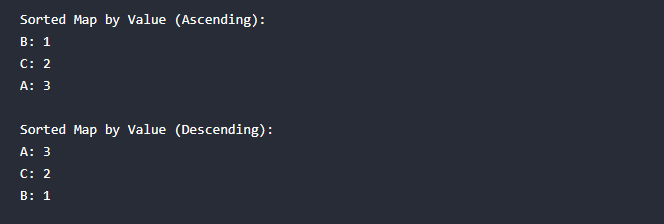
Explanation: The above code sorts a Map by its values using the sort() method. Firstly, it converts the map into a list, and then sorts the list in ascending order by value.
Note: Sorting directly within a map without converting it to a list first is not possible; that is why the sort() method is used. Also, you can use the Collections.reverseOrder() to sort the values in reverse order.
Get 100% Hike!
Master Most in Demand Skills Now!
5. Using collect() and toMap() Method in Java
In this method, we use the Stream API to sort a Map by its values and then collect the sorted values into another Map. This method converts the Map into a stream and then collects the results into a new Map by using the collect() method with Collectors.toMap().
Example:
Output:

Explanation: In the above Java code, a Map is sorted by its values, and then it collects the sorted entries into a new Map. Then it uses the collect() method to put the sorted entries into a new LinkedHashMap, which maintains the order. This method creates a new sorted map without changing the original one.
6. Using Custom Code in Java
This method means writing your own logic to sort a map, instead of using the built-in tools like Stream or Collections.sort(), like in other methods. For example, you can go through the map values, compare them, and arrange them on the basis of your logic. This method is useful when you need a specific sorting method that is not used by Java’s built-in methods.
Example:
Output:

Explanation: In the above Java code, a Map is used to sort its values by using a manual approach. It first converts the map into a list of values. Then, using a loop, it compares and swaps them, which allows you to control the flow of the Map.
Comparison between different Methods for Sorting the Values in a Map
Methods | Time Complexity | Code Complexity | Flexibility | Suitable For |
---|---|---|---|---|
List<Map.Entry<K, V>> and Comparator |
O(n log n) | Moderate | Highly customizable sorting logic | Pre-Java 8 projects or fine-grained control |
Stream API | O(n log n) | Low | Medium – concise but structured | Medium – easy to tweak the comparator |
Lambda Expressions | O(n log n) | Low | High – easily integrate custom logic | Clean and expressive sorting needs |
sort() on List of Entries |
O(n log n) | Low to Moderate | sort() on the List of Entries |
Quick implementations and custom sort orders |
collect() + toMap() |
O(n log n) | Low | Medium – best with LinkedHashMap | One-liners in production or stream pipelines |
Custom Sorting Code (manual implementation) | O(n²) or O(n log n) | High | Very High – full control over logic | Educational purposes or highly specific cases |
Free Online Java Certification Course
This free Java certification course can help you develop the right skills that are essential to establish yourself as a qualified Java professional and get high-paying job opportunities in top MNCs.
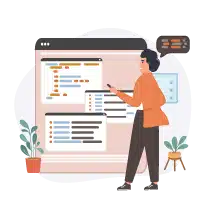
Conclusion
You cannot sort the values of a Map directly in Java, but it can be achieved by using many different techniques. You can sort it using List, Comparator, Stream API, lambda, or collect() methods, depending on your Java version and your choice. Each method has its own advantages and disadvantages. Try to use LinkedHashMap, as it maintains the order of the sorted values.
If you want to learn more about Java, you can refer to our Java Course.