Arithmetic Operators in C are the symbols that are used to perform basic mathematical operations. These operators are easy to use and also support various data types. In this article, we will discuss what arithmetic operators in C are, types of arithmetic operators, precedence and associativity, examples of arithmetic operators in C programming, advantages, and best practices for using arithmetic operators in C.
Table of Contents:
What are Arithmetic Operators in C?
Arithmetic operators in C are the operators that perform arithmetic operations on numeric values. These operators perform calculations such as addition(+), subtraction(-), multiplication(*), division(/), and modulus(%).
Syntax of Arithmetic Operators in C
Arithmetic operators have a very basic syntax in C because the operators are placed between two operands to perform various calculations.
General Syntax:
result = operand1 operator operand2;
Where:
- operand1 and operand2 are the numerical values or variables.
- operator is one of the arithmetic operators (+, -, *, /, %).
- and, result stores the computed value.
Types of Arithmetic Operators in C
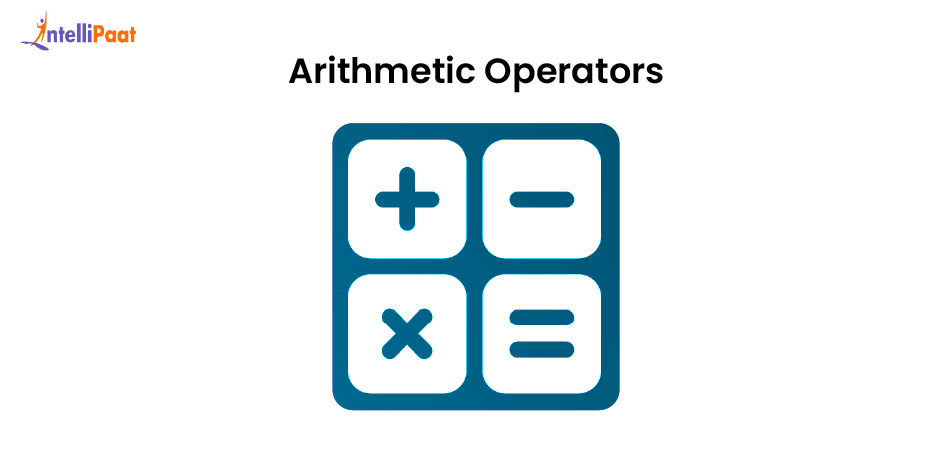
There are five types of arithmetic operators, which are classified on the basis of their operations.
Here is the list of arithmetic operators in C:
Operator Name | Symbol | Description | Syntax |
Addition | + | Adds two numbers | x + y |
Subtraction | – | Subtracts one number from another | x – y |
Multiplication | * | Multiplies two numbers | x * y |
Division | / | Divides one number by another (quotient) | x / y |
Modulus | % | Returns the remainder of integer division | x % y |
Let’s discuss each of the types in brief, with the examples in C.
1. Addition Operator (+)
The addition operator in C is used to add two numbers or operands.
Syntax:
result = operand1 + operand2;
Example:
Output:

In this code, the addition operator adds two integers, x and y, with values 10 and 5, and prints the result 15 as an output to the console.
2. Subtraction Operator (-)
The subtraction operator in C is used to subtract one number or operand from another.
Syntax:
result = operand1 - operand2;
Example:
Output:

In this code, the subtraction operator subtracts two integers, a = 10 and b = 5, and the result, 5, is printed to the console.
3. Multiplication Operator (*)
The multiplication operator in C is used to multiply two numbers or operands.
Syntax:
result = operand1 * operand2;
Example:
Output:

In this code, the multiplication operator multiplies two integers, a and b, with the values of 10 and 5, and the result is printed to the console.
4. Division Operator (/)
The division operator in C is used to divide one number by another. It gives a quotient as a result of the division.
Syntax:
result = operand1 / operand2;
Example:
Output:

In this code, the division operator divides the integer a by b and float x by y, and then the result of both divisions is printed to the console.
5. Modulus Operator (%)
The modulus operator in C is used to return the remainder from the division of two integers.
Syntax:
result = operand1 % operand2;
Example:
Output:

In this code, the modulus operator gives remainder 1 as a result after the division of two integers, a = 10 and b = 3, which is the remainder of that division.
Precedence and Associativity of Arithmetic Operators in C
The operator precedence checks the order in which operations are performed. The operators with higher precedence are evaluated first.
The associativity defines the order of evaluation. The arithmetic operators have left-to-right associativity, which means that operations are evaluated from left to right with operators of the same precedence.
Here is a list of the precedence level and associativity of the arithmetic operators:
Operator | Precedence | Associativity |
* | Highest | Left to Right |
/ | Higher | Left to Right |
% | Higher | Left to Right |
+ | Lower | Left to Right |
– | Lowest | Left to Right |
Examples of Arithmetic Operators in C Programming
Below are a few examples of arithmetic operators in C programming:
1. Calculating the Area of a Circle
This C program uses the multiplication operator for calculating the area of a circle using the formula PI * r * r, by taking the radius input by the user.
Output:

2. Compound Interest Calculation
This C program calculates the compound interest using the formula A = P * (1 + r/100)^t. In this formula, P is the principal, r is the rate, and t is the time. The pow() function is used for exponentiation.
Output:

3. Average of Two Numbers
This C program shows how the average of two numbers is calculated using the formula [(x + y) / 2], and then the result is printed with two decimal places.
Output:

4. Converting Temperature from Celsius to Fahrenheit
This C program converts Celsius to Fahrenheit using the formula F = (C * 9/5) + 32, and then the result is printed with two decimal places.
Output:

5. Even or Odd Number
This C program checks that the number given by the user as an input is even or odd using the modulus operator.
Output:

Advantages of Arithmetic Operators in C
- The arithmetic operators are fast and efficient.
- They have a simple syntax that provides easier calculations.
- It works with various data types, such as int, float, and double, for the calculations.
- Arithmetic operators help to improve the readability.
- It is widely used in applications such as scientific and financial calculations.
Best Practices for Using Arithmetic Operators in C
- You must use the ( ) bracket to make sure that there is the correct order of operations.
- You should always check if the denominator is zero or not before performing division to avoid runtime errors.
- Always be careful while doing integer division operations.
- You must choose proper data types, such as int, float, and double, based on the need for the calculations.
- You should use the modulus operator only with integers, as it does not work with any other data types.
- Always store the results in variables instead of recalculating the same thing many times.
- You must follow the proper and consistent spacing around the operators for better readability.
Conclusion
To conclude, we may refer to the discussion above that the Arithmetic Operators in C are used for calculations. They not only support different types of data, but operators are often used in programs that are scientific, financial, or for general programming. Therefore, with an understanding of precedence, associativity, and best practices, you will be able to perform calculations efficiently and quickly in C programs.
Arithmetic Operators in C – FAQs
Q1. What do arithmetic operators do in C?
The arithmetic operators perform basic mathematical operations.
Q2. Can I use modulus with floating-point numbers?
No, you cannot use them with the modulus operator.
Q3. What will be the result if I divide by zero?
It causes a runtime error or undefined behavior.
Q4. How to perform exponentiation in C?
You can use pow() from <math.h> to perform exponentiation in C.
Q5. Can arithmetic operators be used in if conditions?
Yes, the arithmetic operators can be used in if conditions for decision-making.